Brute Force Lines:
void setup() {
size (500, 500);
background(255);
}
void draw() {
////vertical lines
//line(50, 0, 50, 500);
//line(100, 0, 100, 500);
//line(150, 0, 150, 500);
//line(200, 0, 200, 500);
//line(250, 0, 250, 500);
//line(300, 0, 300, 500);
//line(350, 0, 350, 500);
//line(400, 0, 400, 500);
//line(450, 0, 450, 500);
////horizontal lines
//line(0,50,500,50);
//line(0,100,500,100);
//line(0,150,500,150);
//line(0,200,500,200);
//line(0,250,500,250);
//line(0,300,500,300);
//line(0,350,500,350);
//line(0,400,500,400);
//line(0,450,500,450);
////resize with window
int a =width/5;
int b=height/5;
////vertical lines
line(a, 0, a, height);
line(a/2, 0, a/2, height);
line(a*1.5, 0, a*1.5, height);
line(a*2, 0, a*2, height);
line(a*2.5, 0, a*2.5, height);
line(a*3, 0, a*3, height);
line(a*3.5, 0, a*3.5, height);
line(a*4, 0, a*4, height);
line(a*4.5, 0, a*4.5, height);
////horizontal lines
line(0, b, width, b);
line(0, b/2, width, b/2);
line(0, b*1.5, width, b*1.5);
line(0, b*2, width, b*2);
line(0, b*2.5, width, b*2.5);
line(0, b*3, width, b*3);
line(0, b*3.5, width, b*3.5);
line(0, b*4, width, b*4);
line(0, b*4.5, width, b*4.5);
}
Brute Force Rectangles:
void setup () {
size (500, 500);
background(255);
}
void draw () {
//column1
rect(0, 0, 50, 50);
rect(0, 50, 50, 50);
rect(0, 100, 50, 50);
rect(0, 150, 50, 50);
rect(0, 200, 50, 50);
rect(0, 250, 50, 50);
rect(0, 300, 50, 50);
rect(0, 350, 50, 50);
rect(0, 400, 50, 50);
rect(0, 450, 50, 50);
//column2
rect(50, 0, 50, 50);
rect(50, 50, 50, 50);
rect(50, 100, 50, 50);
rect(50, 150, 50, 50);
rect(50, 200, 50, 50);
rect(50, 250, 50, 50);
rect(50, 300, 50, 50);
rect(50, 350, 50, 50);
rect(50, 400, 50, 50);
rect(50, 450, 50, 50);
//column3
rect(100, 0, 50, 50);
rect(100, 50, 50, 50);
rect(100, 100, 50, 50);
rect(100, 150, 50, 50);
rect(100, 200, 50, 50);
rect(100, 250, 50, 50);
rect(100, 300, 50, 50);
rect(100, 350, 50, 50);
rect(100, 400, 50, 50);
rect(100, 450, 50, 50);
//column4
rect(150, 0, 50, 50);
rect(150, 50, 50, 50);
rect(150, 100, 50, 50);
rect(150, 150, 50, 50);
rect(150, 200, 50, 50);
rect(150, 250, 50, 50);
rect(150, 300, 50, 50);
rect(150, 350, 50, 50);
rect(150, 400, 50, 50);
rect(150, 450, 50, 50);
//column5
rect(200, 0, 50, 50);
rect(200, 50, 50, 50);
rect(200, 100, 50, 50);
rect(200, 150, 50, 50);
rect(200, 200, 50, 50);
rect(200, 250, 50, 50);
rect(200, 300, 50, 50);
rect(200, 350, 50, 50);
rect(200, 400, 50, 50);
rect(200, 450, 50, 50);
//column6
rect(250, 0, 50, 50);
rect(250, 50, 50, 50);
rect(250, 100, 50, 50);
rect(250, 150, 50, 50);
rect(250, 200, 50, 50);
rect(250, 250, 50, 50);
rect(250, 300, 50, 50);
rect(250, 350, 50, 50);
rect(250, 400, 50, 50);
rect(250, 450, 50, 50);
//column7
rect(300, 0, 50, 50);
rect(300, 50, 50, 50);
rect(300, 100, 50, 50);
rect(300, 150, 50, 50);
rect(300, 200, 50, 50);
rect(300, 250, 50, 50);
rect(300, 300, 50, 50);
rect(300, 350, 50, 50);
rect(300, 400, 50, 50);
rect(300, 450, 50, 50);
//column8
rect(350, 0, 50, 50);
rect(350, 50, 50, 50);
rect(350, 100, 50, 50);
rect(350, 150, 50, 50);
rect(350, 200, 50, 50);
rect(350, 250, 50, 50);
rect(350, 300, 50, 50);
rect(350, 350, 50, 50);
rect(350, 400, 50, 50);
rect(350, 450, 50, 50);
//column9
rect(400, 0, 50, 50);
rect(400, 50, 50, 50);
rect(400, 100, 50, 50);
rect(400, 150, 50, 50);
rect(400, 200, 50, 50);
rect(400, 250, 50, 50);
rect(400, 300, 50, 50);
rect(400, 350, 50, 50);
rect(400, 400, 50, 50);
rect(400, 450, 50, 50);
//column10
rect(450, 0, 50, 50);
rect(450, 50, 50, 50);
rect(450, 100, 50, 50);
rect(450, 150, 50, 50);
rect(450, 200, 50, 50);
rect(450, 250, 50, 50);
rect(450, 300, 50, 50);
rect(450, 350, 50, 50);
rect(450, 400, 50, 50);
rect(450, 450, 50, 50);
}
For Loop Lines:
void setup() {
size (500,500);
background(255);
}
void draw() {
//horizontal
for (int a=width/10; a<width; a+=width/10) {
line(0,a,width,a);
}
//vertical
for (int b=height/10; b<height; b+=height/10) {
line(b,0,b,height);
}
}
For Loop Rectangles:
void setup() {
size(500, 500);
background(255);
}
void draw() {
for (int y = 0; y < width; y+= 50) {
for (int x = 0; x <= height; x += 50)
rect (x, y, height/10, width/10);
}
}
Array Lines:
int[] coordinates = { 50, 100, 150, 200, 250, 300, 350, 400, 450};
void setup() {
size (500, 500);
background(255);
}
void draw() {
//horizontal
for (int y=0; y<coordinates.length; y++) {
line (0, coordinates[y], width, coordinates[y]);
}
//vertical
for (int x=0; x<coordinates.length; x++) {
line (coordinates[x], 0, coordinates[x], height);
}
}
Array Rectangles:
int[] coordinates = {0, 50, 100, 150, 200, 250, 300, 350, 400, 450};
void setup() {
size(500, 500);
background(255);
}
void draw() {
for (int y = 0; y <coordinates.length; y++) {
for (int x = 0; x <coordinates.length; x++)
rect (coordinates[x], coordinates[y], height/10, width/10);
}
}
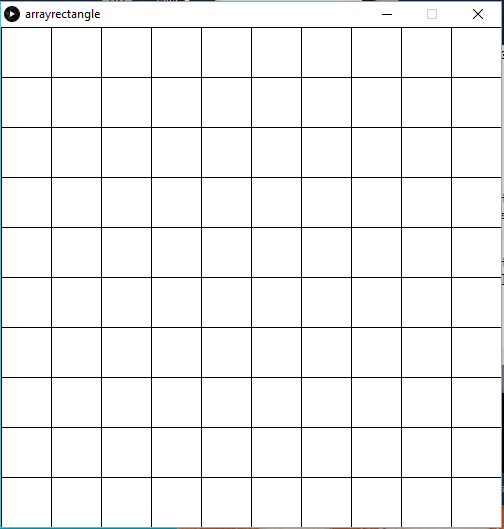